- An introduction to Web Scraping with Python and Azure Functions - PyLadies Amsterdam Online 26 May from 4:30pm UTC to 6pm UTC, 2021.
- Nov 08, 2018 There are different ways of scraping web pages using python. In my previous article, I gave an introduction to web scraping by using the libraries:requests and BeautifulSoup. However, many web pages are dynamic and use JavaScript to load their content. These websites often require a different approach to gather the data.
On my machine, I happen to have Python 2 and Python 3 installed, so I can create a Notebook that uses either of these. For simplicity’s sake, let’s choose Python 3. Your web page should now look like this: Naming. You will notice that at the top of the page is the word Untitled. This is the title for the page and the name of your Notebook.
Web scraping is a method of extracting and restructuring information from web pages. This workshop will introduce basic techniques for web scraping using the popular Python libraries BeautifulSoup and Requests. Participants will practice accessing websites, parsing information, and storing data in a CSV file. This workshop is intended for social scientists who are new to web scraping, but have some familiarity with Python or have attended the Introduction to Python workshop.
Workshop Preparation
Computers with Python pre-loaded are available on a first-come, first-served basis. If you with to use your own laptop, please install the Anaconda distribution of Python 3.6.
If you are having trouble installing this version of Python, please contact data science services at help@iq.harvard.edu.
Hosted by Data Science Services at Harvard’s Institute for Quantitative Social Science. This workshop is free for Harvard and MIT affiliates.
Location: Harvard Campus, K018, CGIS Knafel building, concourse level
Register
Contact:researchconsulting_support@help.hmdc.harvard.edu
Introduction
In this post, we'll cover how to scrape Newegg using python, lxml and requests. Python is a great language that anyone can pick up quickly and I believe it's also one of the more readable languages, where you can quickly scan the code to determine what it is doing.
Just look at this loop with auto incrementing index:
We'll scrape Newegg with the use case of monitoring prices and inventory, especially the RTX 3080 and RTX 3090.
Setting up
We're going to work in a virtual python environment which helps us address dependencies and versions separately for each application / project. Let's create a virtual environment in our home directory and install the dependencies we need.
Make sure you are running at least python 3.6, 3.5 is end of support.
Let's create the following folders and files.
We created a __main__.py
file, this lets us run the Newegg scraper with the following command (nothing should happen right now):
Crawling the content
We need to write code that can crawl the content, by crawl I mean fetch or download the HTML from the target website. Our first target is Newegg, this website doesn't seem to require javascript for the data we need. We'll get into rendering javascript in a future post that covers headless scraping using requests-html on Google Places.
Open core/crawler.py
which we created earlier. Now, we'll begin by requesting the HTML content from Newegg's domain.
In newegg/__main__.py
we can import crawler and the code above will execute.
Remember you can execute and test your code with the previous python command in your terminal (must be run in the root folder ~/intro-web-scraping
).
It looks like the request succeeded, the status code should of been printed to your terminal with a success of 200
.Let's clean up the code to make it reusable and define a function for returning the response text.
In core/crawler.py
we'll define a crawl_html
function (we want to reuse it and this lets us redefine where the HTML comes from in the future).
In newegg/__main__.py
we'll use the function, you can run it and see the HTML being printed. We use an uppercased variable NEWEGG_URL
to define a constant - something that shouldn't change.
Scraping the data we need
Now that we have access to the HTML content from Newegg, we want a way to pull out stock information and price for the RTX 3080 and RTX 3090.Let's find the page from Newegg that has that information first.
Navigate to https://www.newegg.com/p/pl?N=100007709%20601357282 in your browser and you'll see we have filters applied for RTX 30 series.
We'll take that path and append it to our NEWEGG_URL
. We do this using f-strings in python, which is a way to interpolate variables in strings.
From this URL we can start scraping the data we need. Let's start by creating a few useful functions in the file core/scraping.py
. These functions wrap lxml and handle some of the type conversions to make it easier for us to work with.
Finding the data
We'll first try to get the prices with XPath. I highly recommend you use XPath instead of CSS selectors which is much more declarative and more expressive, you can use this simple cheat sheet for quickly finding out how to specify selectors. A more in-depth guide can be found from librarycarpentry.
Open your chrome browser and visit the crawl url we defined earlier: https://www.newegg.com/p/pl?N=100007709%20601357282.
Press F12 on your keyboard or open the developer console by right-clicking one of the prices on the page and selecting inspect
.
Using XPath
We'll use the inspector and practice our XPath to figure out how to get all prices on the page (there are 29 items listed). This selector: //li[contains(@class, 'price-current')]
grabs all relevant prices.
With the selector in hand, let's modify our newegg/__main__.py
entry file by adding a new function to grab the prices.
We should see output like the following.
Let's clean this extra HTML entity appearing at the end of our prices with a utility function. We'll make use of re
for regex and unescape
from html module to cleanup our data. We need to check if the input contains numbers in order to account for the COMING SOON
labels. We'll keep this logic encapsulated in our get_rtx_prices
by mapping over each item and then converting it back to a list (map
returns an object iterator).
Let's grab the item names.
We also want the link to the item.
More complex XPath
Next we want the stock information (out of stock or in stock). To do this we need to add another function called get_children_text
to core/scraper.py
. This will allow us to specify a parent selector and a child selector, which will return the first child that matches. If our parent selector has many matches it will try to find a matching child and if it does not find one it will return None
. In our case we have many parent matches but some of them may not contain the OUT OF STOCK
element.
In core/scraper.py
add the new function.
Back in newegg/__main__.py
we can add the stock selector.
We also want the product id, having this can help us track changes to the product in the future. Here's how we can find the item id from the page.
If you notice on the highlighted lines below, you can see we added another function to our scraper. Because we are using the text()
function of XPath, we are asking for the text node which ignores the other strong
label node in the tree seen in the screenshot above.
Let's add get_nodes
to our core/scraper.py
module.
Our final output structure
Let's put it all together now to generate the final structure for our output which will contain basic stock information, price, product name, product id and product link.
This is what our newegg/__main__.py
should look like now.
Ommitted some of the results for readability, but the output should total 29 products as of this post.
Saving our data
With our data in hand, we can quickly save it for analysis later - it's not hard to imagine what else is possible when you have the data you want. We could monitor the price changes of these items, their stock status or when new items are added.
Let's add two csv utility functions to our core/utils.py
file. We will write one to tansform our scraped output to proper csv lines and another to write the csv output.

We can use it in our newegg/__main__.py
file and just save the output we receive from get_rtx_items
. First import the utils at the top of the file.
Now let's use our utility function at the bottom of our Newegg scraper to save the output and complete the full web scraping cycle - crawling, scraping and saving the output.
Checking the output
We can open the csv file to view the output which is saved in the folder we created at the beginning ~/intro-web-scraping
.
Wrapping up
From this guide we should have learned most of what I believe is the web scraping basics:
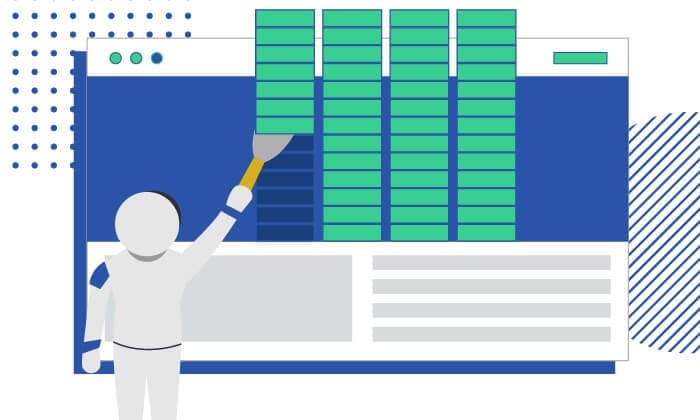
Introduction To Web Scraping With Python Pdf

- Crawling content (using requests)
- Scraping relevant data (lxml and XPath)
- Saving the output (writing to a csv file)
What we didn't cover:
- Headers
- Proxies (residential, data center, tor)
- Headless browsers
- Bot detection (fingerprinting)
- Throttling
- Captcha (recaptcha, image based input)
In a future post, we will scrape a website which requires javascript rendering and we'll make use of the requests-html python library to render the page and execute javascript.
Introduction To Web Scraping With Python Answers
Hopefully you'll find this post enlightening as web scraping has some really creative use cases that are not so obvious. Till next time!
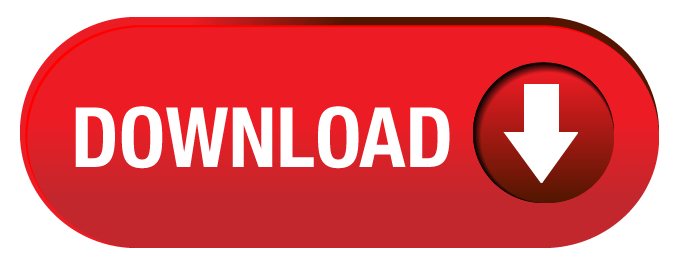